create pay now order
method 1​
make a server-to-server call to create a payment order.
endpoint: POST {{API_BASE_URL}}/paynow/orders
here is the workflow diagram showing the whole payment process.
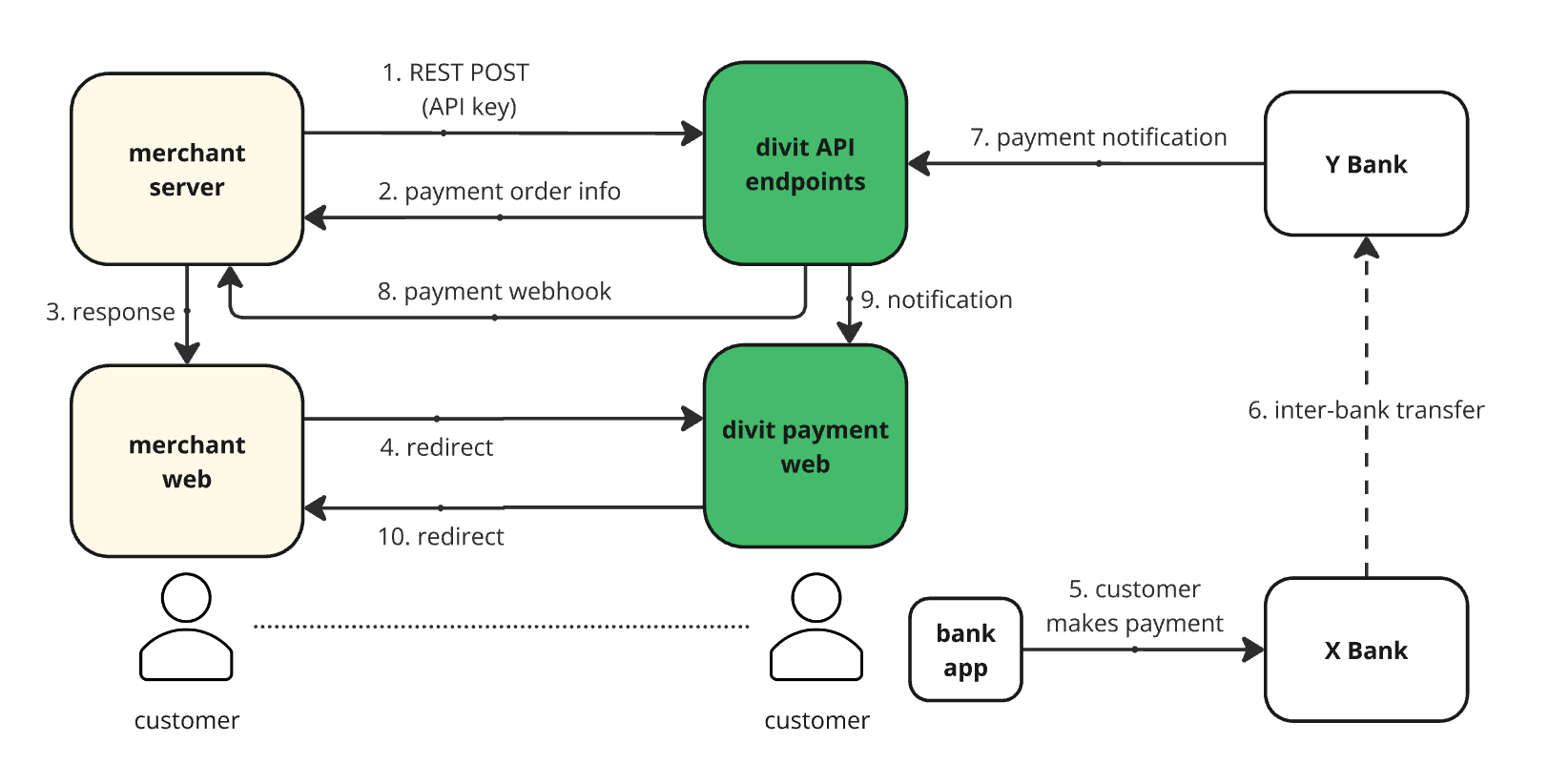
steps:
- merchant server POST a create order request to divit server endpoint (step 1)
- divit response with JSON object contains redirect URI and access token (step 2)
- merchant website redirect customer to divit payment screen (step 3 - 4)
- customer makes a payment, bank transfer money to divit account (step 5 - 6)
- bank send real-time credit notification to divit server (step 7)
- divit sends webhook call to merchant server, and push notification to divit payment screen (step 8 - 9)
- divit payment screen redirect user back to merchant website (step 10)
order creation API​
to create a payment order, you'll need to supply both the order and customer information. ensure you include the API key in the request header for authentication. if you wish for your customers to join the divit reward campaign or lucky draw, you must also provide some customer contact information.
caution
all monetary values are in integer format. So, $987.50 = 98750
var data = JSON.stringify({
"order": {
"totalAmount": {
"amount": 0,
"currency": "string"
},
"webhookSuccess": "string", // URL for redirecting customer to after successful payment
"webhookFailure": "string", // URL for redirecting customer to after failure/cancelled payment
"webhookEvents": "string", // URL receives webhook events
"expiredAt": 1696654130, // unix time
"merchantRef": "string" // your reference ID that customer will see on the payment screen
"merchantUniqueOrderID": "string", // in case you have another unique id referring to the payment
"language": "en", // we supports "en", "zh-hk", "zh-cn"
...<orderItems: check 'order item details' section>...,
},
"customer": {
"firstName": "string",
"lastName": "string",
"email": "[email protected]",
"tel": "string",
"country": "HK", // ISO 3166-1 alpha-2 country code
"language": "en"
}
});
var xhr = new XMLHttpRequest();
xhr.withCredentials = true;
xhr.addEventListener("readystatechange", function() {
if(this.readyState === 4) {
console.log(this.responseText);
}
});
xhr.open("POST", "{{API_BASE_URL}}/paynow/orders");
xhr.setRequestHeader("api-key", "{{API_KEY}}"); // e.g. dvt_rREeGJ4FXp69nQEJ7Arq08stvtacFyPrTzKr
xhr.setRequestHeader("Content-Type", "application/json");
xhr.send(data);
order item details​
- retail industry
it is a good practice to send some of the order items information to us, so divit support will quickly get into details about your customer's order
productID
is your own SKU that is attached to the product.productTitle
usually the product nameqty
how many items of the SKU did the user orderpics
any product images to give the user a visual representation of the order
{
...,
"orderType": "retail",
"orderItems": [
{
"itemType": "retail",
"itemData": {
"productID": "string",
"productTitle": "string",
"qty": 0,
"pics": {}
}
}
],
...
}
create order response​
after creating the order, you will receive the redirectURI
together with a token. the token is used to claim back the order created by the backend, linking the purchase session together.
redirect the user to divit with the URI value and append token
as the parameter.
{
"code": 0,
"message": "OK",
"data": {
"redirectURI": "https://sandbox-consumer.divit.dev/paynow/orders/956db0a3-68b1-420c-9df9-3ec5d77136b9/fast?merchant=<merchant name>&mRef=<merchant code>",
"orderID": "956db0a3-68b1-420c-9df9-3ec5d77136b9", // divit order ID {{DIVIT_ORDER_ID}}
"token": "eyJhbGciOiJSUzI1NiIsInR5cCI6IkpXVCJ9.eyJleHAiOjE2NDQ2NzU1NjYsIm9yZCI6Ijk1NmRiMGEzLTY4YjEtNDIwYy05ZGY5LTNlYzVkNzcxMzZiOSIsIm9yaWdfaWF0IjoxNjQ0NjcxOTY2LCJzY29wZSI6InRtcGVuZHVzZXIiLCJzdWIiOiJlYmZmYWZkYS0yNmMwLTRlYzAtODAzNS01MTgzYmYyMjdiMzYifQ.OW4dLVPeoXYvRnqoeGAlKZLTJ2s0ocYNy7DJCvefCTOPue6LPXd08ZdEzFd7MEdOvTpVro9a3J26TvQ22sBEDNIA6DHLTb6wHRi9Bl2bUzRAwrsvoS8w9LUL9VAXAH9qs9kE2Se5hEYGLfAuFSjQ-dBaQ2w_eZpkK3SHEw2SRd_lmpTO76WIN7UqImX35yttWa2p9FPtKFbIoLIBsF3N1xLQveEzl3A_hiDp5asuryDVl73PTy5f01GrDoUS6SekSaVEVOMuPFhBv9MZPEet91_Oe0jrcTqgczxFBY9otODurUJcwzw5LhhwDOm2d-5YYVYzryhr5nHLib_-6TAxJA"
}
}
redirect user​
almost done! just redirect your user to the supplied URL and remember to append the token
parameter, we will take it from there. now you can sit back and wait for our webhookEvents
call.
function pageRedirect() {
window.location.replace("https://sandbox-consumer.divit.dev/paynow/order/956db0a3-68b1-420c-9df9-3ec5d77136b9/fast?merchant=<merchant name>&mRef=<merchant code>&token=<token>");
}
payment screen​
the redirect link will bring your customer to our payment screen
web-to-app payment​
divit supports web-to-app (app-to-app) payment. we can open the banking app and have all the payment information ready by one click on the payment screen.