create direct payment link
method 2​
this is a direct payment method that using divit public endpoint.
endpoint: GET {{API_BASE_URL}}/directpay/c/{{merchantID}}/{{branchID}}/fps/act
here is the workflow diagram showing the whole payment process.
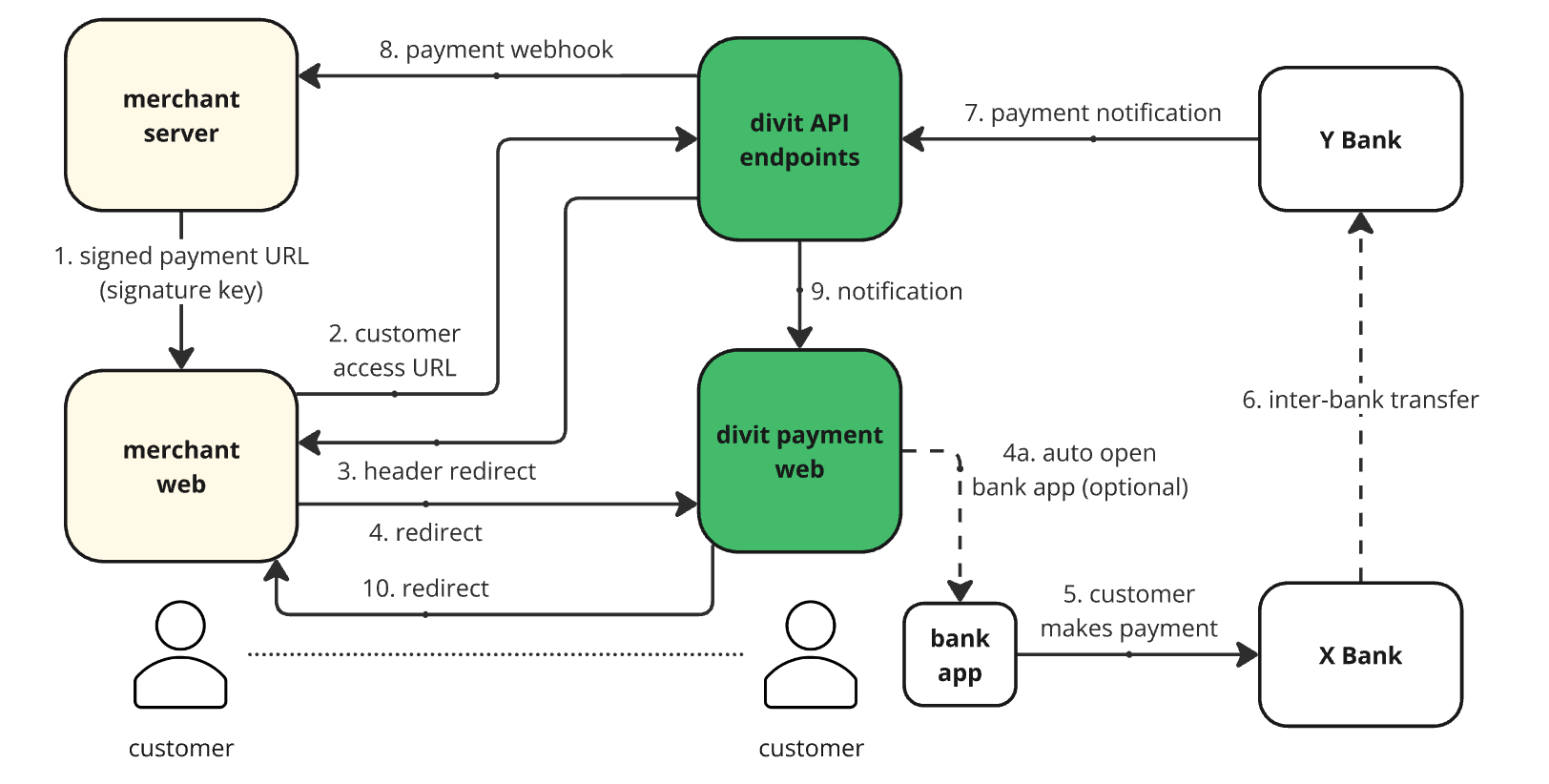
steps:
- merchant server create signed URL and render it on the merchant website (step 1)
- customer clicks on the link (step 2)
- divit server creates payment record and redirect user to the payment screen (step 3 - 4)
- customer makes a payment, bank transfer money to divit account (step 5 - 6)
- bank send real-time credit notification to divit server (step 7)
- divit sends webhook call to merchant server, and push notification to divit payment screen (step 8 - 9)
- divit payment screen redirect user back to merchant website (step 10)
configuration​
you need to predefine the webhook endpoint and redirect URLs at the branch details page of the admin-portal.
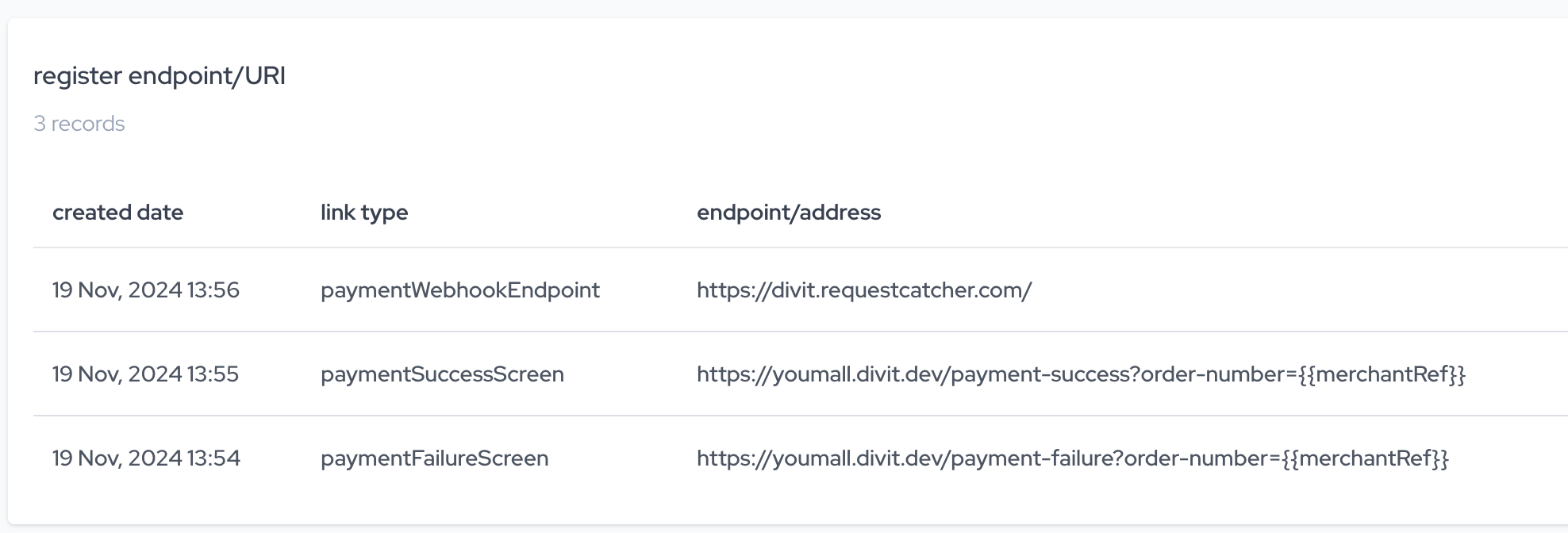
link type | explanation |
---|---|
paymentWebhookEndpoint | your server endpoint to receive our webhook events. details here |
paymentSuccessScreen | when payment is complete, we will redirect customer to this URL |
paymentFailureScreen | when payment is cancelled, we will redirect customer to this URL |
constructing payment link​
to construct the payment link, you will need to get 3 pieces of information from divit admin-portal:
merchantID
: your merchant account IDbranchID
: each merchant can have multiple branches, if you are freshly signed up, a default branch has already been created for you.- signature key: find the value at the corresponding branch details page at the admin-portal
constructs the payment link by providing the following parameters:
amount
: payment amountcurrency
: currencyexpiredAt
: the unix time that the payment will be expired, e.g. 86400 for 1 day of expirymerchantRef
: unique reference of your order
signs the concatenated string of parameters and a timestamp with the following logic:
// the signature-key should be kept in server-side,
// the following logic should not be run on the front-end, this is just for demo purpose.
//
const signatureKey = `${SIGNATURE KEY}`;
const merchantID = `${YOUR_MERCHANT_ID}`;
const branchID = `${YOUR_BRANCH_ID}`;
const timestamp = 1736845297;
const stringTobeSigned = `/directpay/c/${merchantID}/${branchID}/fps/act?amount=1200¤cy=HKD&expiredAt=1736848897&merchantRef=BspR8bFE`;
let hmac = CryptoJS.algo.HMAC.create(CryptoJS.algo.SHA256, signatureKey);
hmac.update(`${timestamp}:${stringTobeSigned}`);
const signature = hmac.finalize().toString(CryptoJS.enc.Base64);
const host = "{{API_BASE_URL}}";
const actionLink = `${host}${stringTobeSigned}&signature=${encodeURIComponent(signature)}×tamp=${timestamp}`;
caution
the sorting of the parameters will affect the signature output. please make sure they are arranged correctly when you calculating the signature
sample payment link​
<a href="https://sandbox-api.divit.dev/directpay/c/4303c954-afbf-463c-ae37-bdcff2ac106a/69a14250-5459-4980-b47b-2096e19c5087/fps/act?amount=1200¤cy=HKD&expiredAt=1736848897&merchantRef=BspR8bFE&signature=NrrFakSrjZGF6Bg7rhNfyqfCkLjfTOvt%2Bcvf0Rbq5gM%3D×tamp=1736845297">pay now</a>
payment screen​
clicking the payment link will call our server endpoint to create a payment request record instantly, it will then redirect your customer to our payment screen
open the bank app directly​
divit facilitates web-to-app (app-to-app) payments, allowing you to open a banking app with all payment information pre-filled with just one click from the payment screen.
info
using this method 2 request, you can redirect users to their bank's app instead of displaying our payment screen. please contact our support team for more details.
error response​
since the payment link access our public api-endpoint, the error message will be returned as a plain text json message, e.g.
{"code":1013,"message":"failed to load merchant data","errorData":"sql: no rows in result set"}